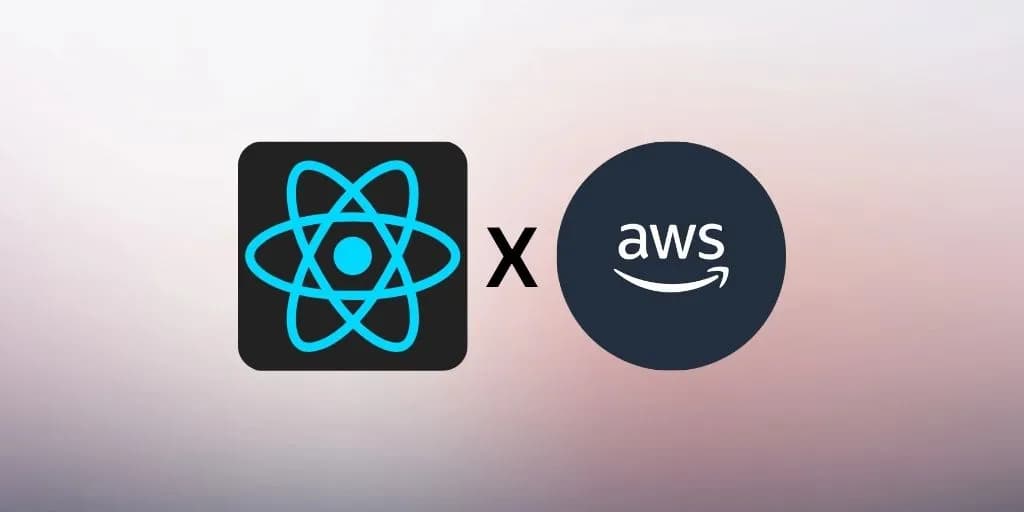
Step-by-Step Guide: How to Deploy a React App on AWS
Deploying a React application on Amazon Web Services (AWS) is a robust way to host your web app. In this guide, we will walk you through the steps required to get your React app up and running on AWS. By the end of this tutorial, you will have a live React application hosted on AWS.
Prerequisites
Before we begin, make sure you have the following installed on your local machine:
- Node.js and npm
- Git
- AWS CLI
Step 1: Create a React App
If you don't already have a React app, you can create one using the following command:
npx create-react-app my-react-app
cd my-react-app
This will create a new React application in a directory called my-react-app
.
Step 2: Initialize a Git Repository
Navigate to your React app directory and initialize a Git repository:
git init
git add .
git commit -m "Initial commit"
Step 3: Set Up AWS Account
Log in to your AWS account and create a new IAM user with programmatic access. Attach the "AmazonS3FullAccess" policy to this user. Note the access key ID and secret access key, as you will need them later.
Step 4: Configure AWS CLI
Configure the AWS CLI with your access key ID and secret access key:
aws configure
Follow the prompts to enter your AWS credentials and set the default region.
Step 5: Create an S3 Bucket
Create a new S3 bucket to host your React app. You can do this from the AWS Management Console or using the AWS CLI:
aws s3 mb s3://my-react-app-bucket
Replace my-react-app-bucket
with a unique name for your S3 bucket.
Step 6: Build Your React App
Build your React app for production:
npm run build
This will create a build
directory with the production build of your app.
Step 7: Deploy to S3
Deploy your React app to the S3 bucket using the AWS CLI:
aws s3 sync build/ s3://my-react-app-bucket --delete
Replace my-react-app-bucket
with the name of your S3 bucket.
Step 8: Configure S3 Bucket for Static Website Hosting
Configure your S3 bucket to host a static website. You can do this from the AWS Management Console or using the AWS CLI:
aws s3 website s3://my-react-app-bucket/ --index-document index.html --error-document index.html
Replace my-react-app-bucket
with the name of your S3 bucket.
Step 9: Set Bucket Policy
Set the bucket policy to make your S3 bucket publicly accessible. Create a bucket-policy.json
file with the following content:
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "PublicReadGetObject",
"Effect": "Allow",
"Principal": "*",
"Action": "s3:GetObject",
"Resource": "arn:aws:s3:::my-react-app-bucket/*"
}
]
}
Replace my-react-app-bucket
with the name of your S3 bucket. Apply the bucket policy using the AWS CLI:
aws s3api put-bucket-policy --bucket my-react-app-bucket --policy file://bucket-policy.json
Step 10: Open Your App
You can open your deployed React app in your browser by navigating to the S3 bucket URL:
http://my-react-app-bucket.s3-website-<region>.amazonaws.com
Replace <region>
with the AWS region where your bucket is hosted.
Conclusion
Congratulations! You have successfully deployed your React app on AWS. In this guide, we covered the steps to create a React app, initialize a Git repository, set up an AWS account, configure the AWS CLI, create an S3 bucket, build your React app, deploy your app to S3, configure the S3 bucket for static website hosting, set the bucket policy, and open your app. Now you can share your live React application with the world.
If you encounter any issues or have any questions, feel free to refer to the AWS documentation or the React documentation. Happy coding!
Recent Posts
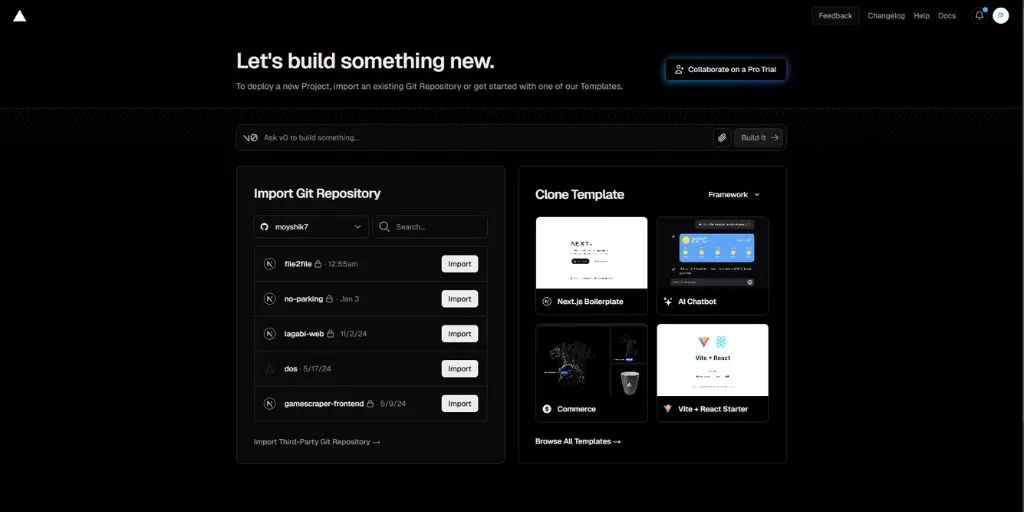
Hosting E-commerce Websites on Vercel
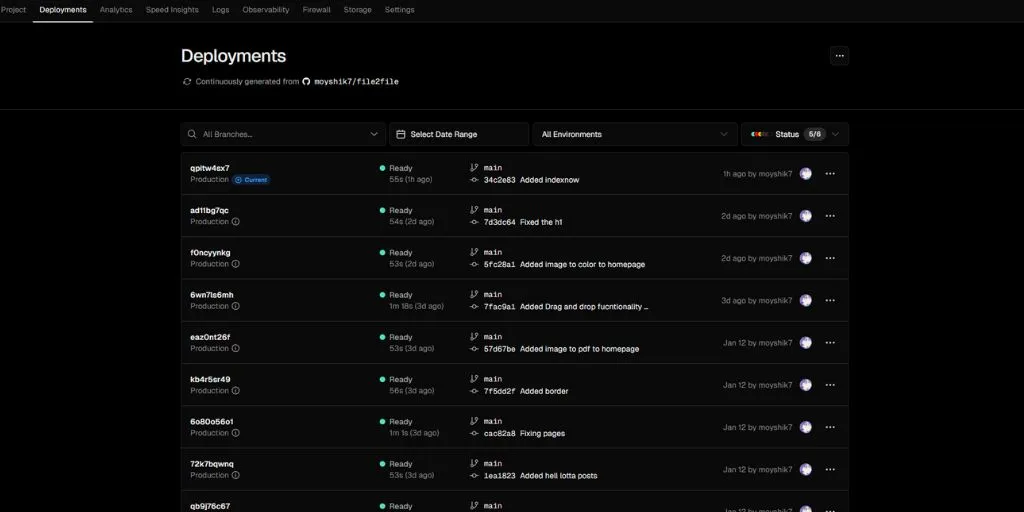
Vercel vs Netlify: Which is Better?
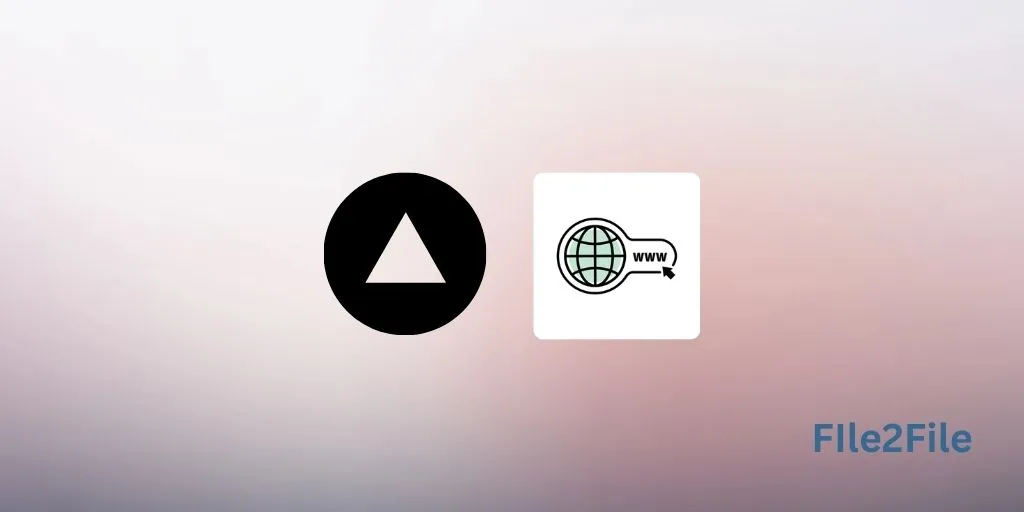
How to Set Up Custom Domains on Vercel: A Compr...
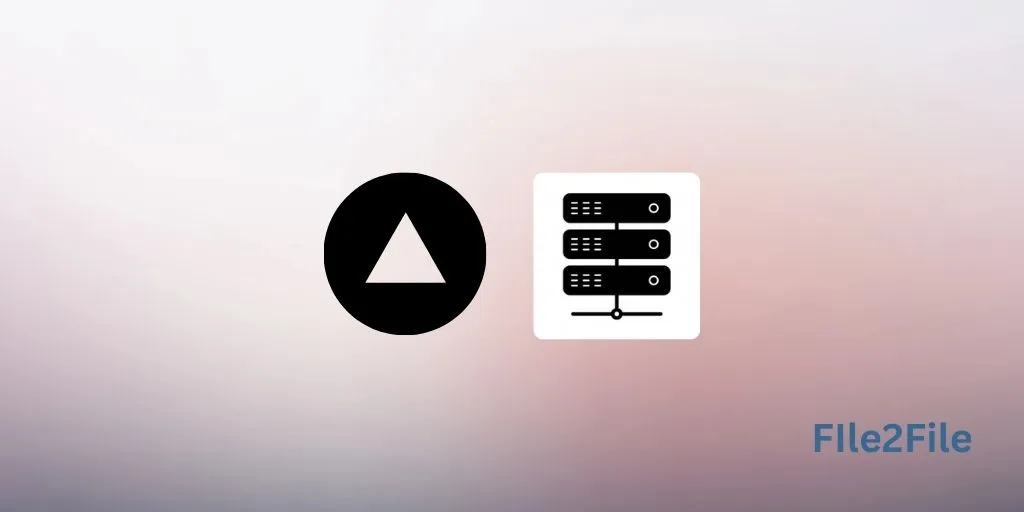
Getting Started with Vercel Hosting: A Step-by-...
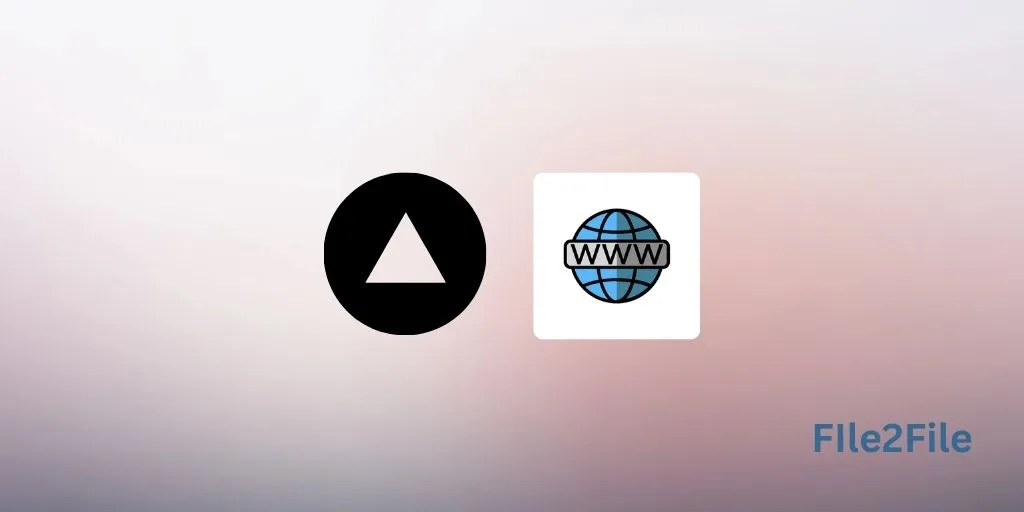
Como Migrar um Aplicativo para Vercel
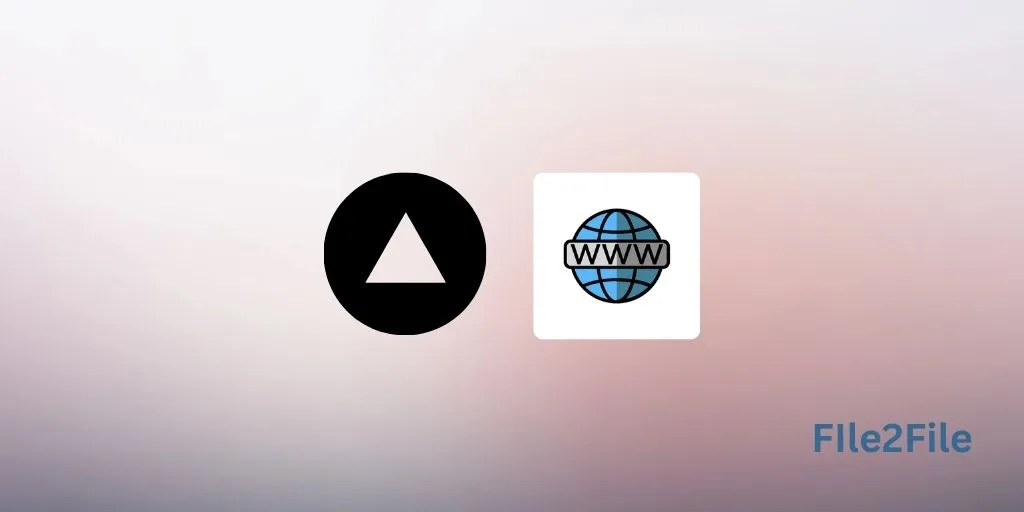
How to Migrate an App to Vercel
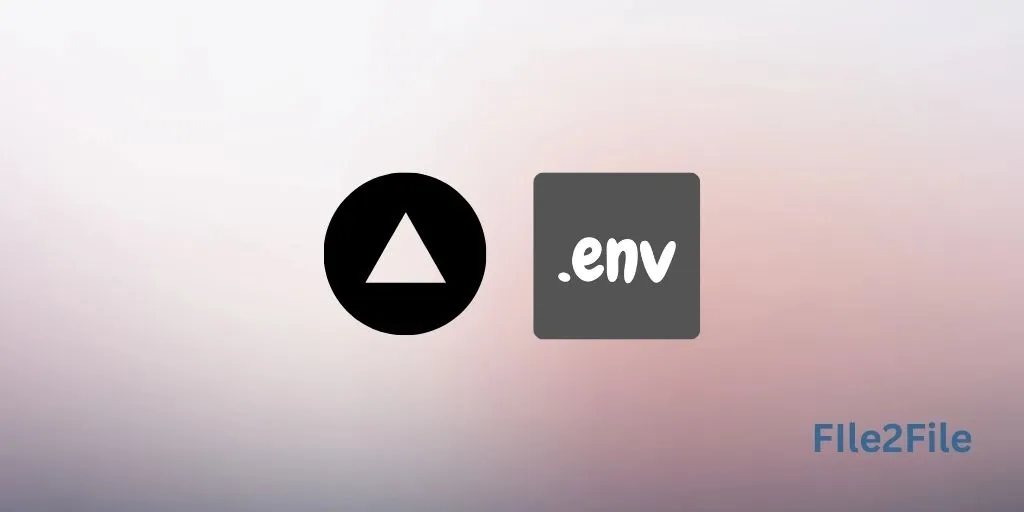
How to Manage Environment Variables on Vercel f...
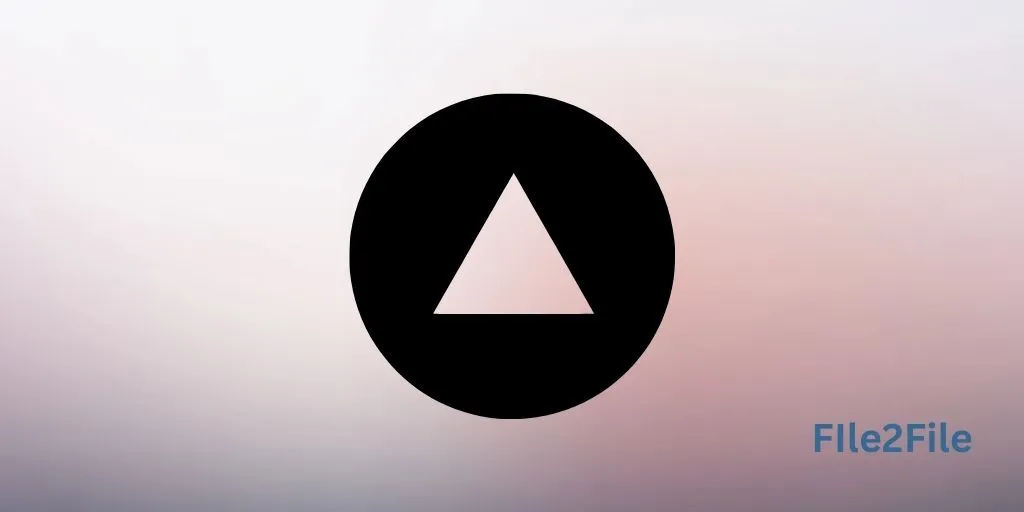