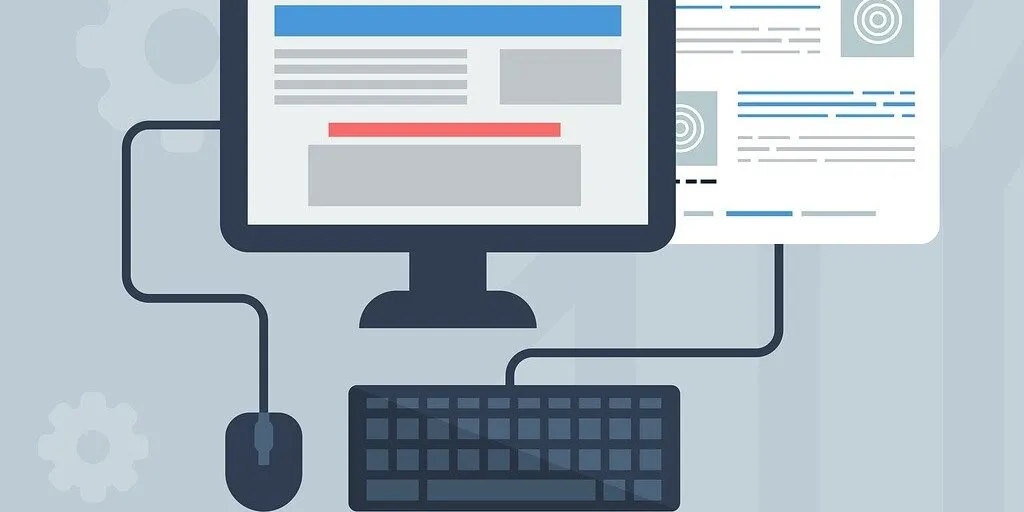
Why You Should Have a Sitemap and How to Generate One by File2File
What is a Sitemap?
A sitemap is an XML file that lists all the pages on your website, helping search engines discover and index your content efficiently. It provides metadata like update frequency and priority for each URL.
Why You Should Have a Sitemap
- Boosts SEO: Ensures all your pages are discoverable by search engines.
- Improves Indexing: Highlights important pages and newly added content.
- Enhances Crawl Efficiency: Guides crawlers, especially for large websites.
How to Generate a Sitemap in Popular Frameworks
1. Node.js:
- Use the `sitemap` package:
const { SitemapStream, streamToPromise } = require('sitemap');
app.get('/sitemap.xml', async (req, res) => {
const smStream = new SitemapStream({ hostname: 'https://example.com' });
smStream.write({ url: '/', changefreq: 'daily', priority: 1.0 });
smStream.end();
const sitemap = await streamToPromise(smStream);
res.type('application/xml').send(sitemap.toString());
});
2. React:
- Generate a static
sitemap.xml
and place it in thepublic
folder.
3. Vue:
- Use a plugin like
vue-cli-plugin-sitemap
or manually create an XML file.
4. Next.js:
- Create a dynamic API route:
export default function handler(req, res) {
res.setHeader('Content-Type', 'application/xml');
res.send(`
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
<url><loc>https://example.com/</loc></url>
</urlset>
`);
}
5. WordPress:
- Use Yoast SEO or other sitemap plugins to automate generation.
6. Django:
- Use the
django.contrib.sitemaps
framework:
from django.contrib.sitemaps import Sitemap
class StaticViewSitemap(Sitemap):
def items(self):
return ['home', 'about']
7. Flask:
- Manually serve XML:
@app.route('/sitemap.xml')
def sitemap():
return Response(
"""<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
<url><loc>https://example.com/</loc></url>
</urlset>""",
mimetype='application/xml'
)
By creating sitemaps and robots.txt files, you ensure your website is both user- and search-engine-friendly, boosting visibility and performance.
Recent Posts
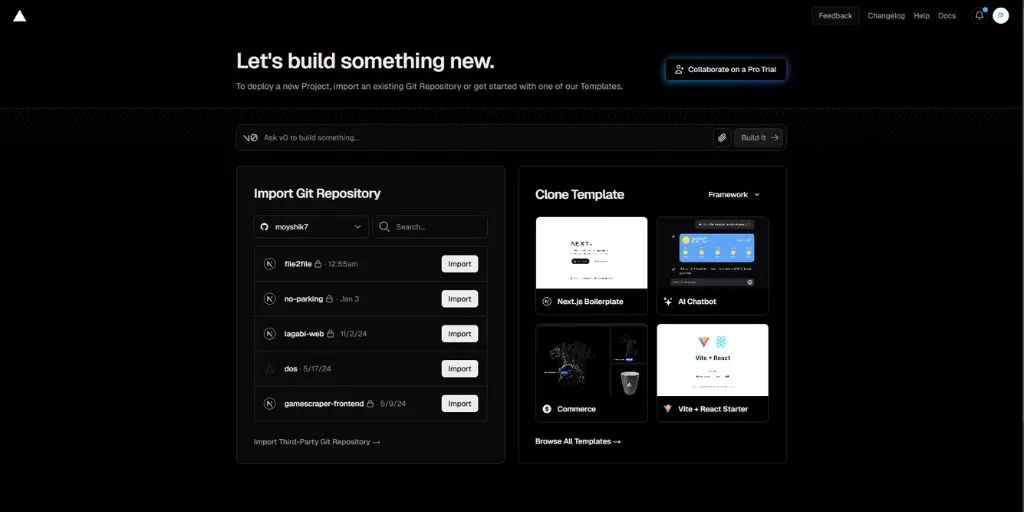
Hosting E-commerce Websites on Vercel
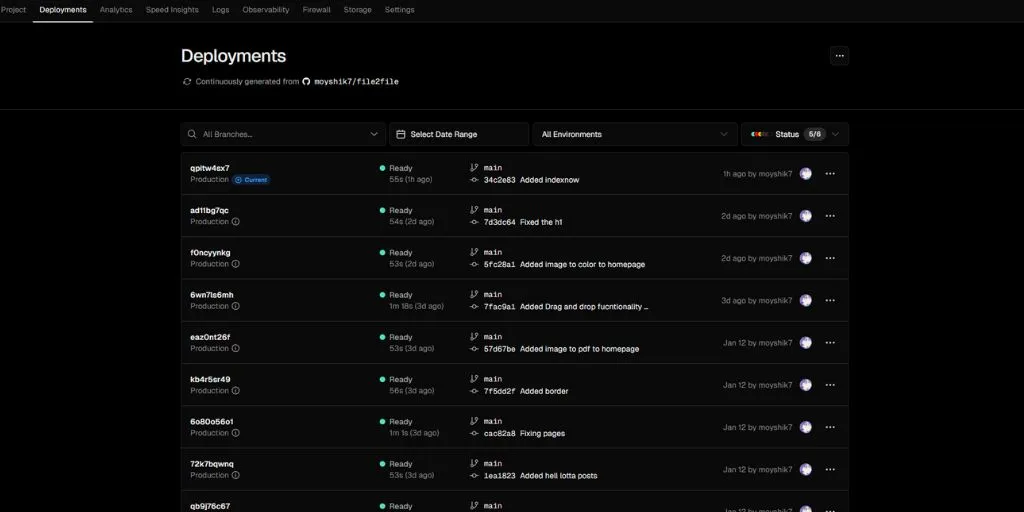
Vercel vs Netlify: Which is Better?
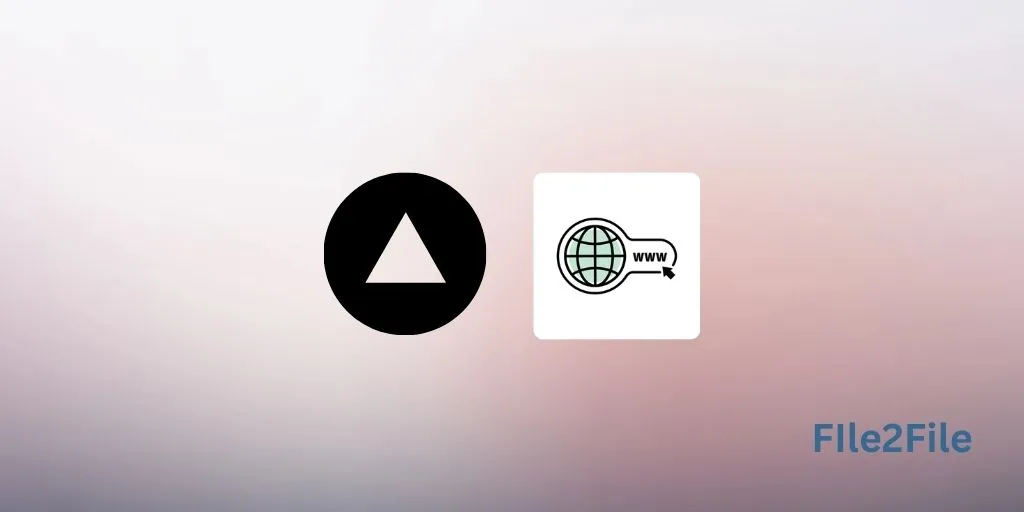
How to Set Up Custom Domains on Vercel: A Compr...
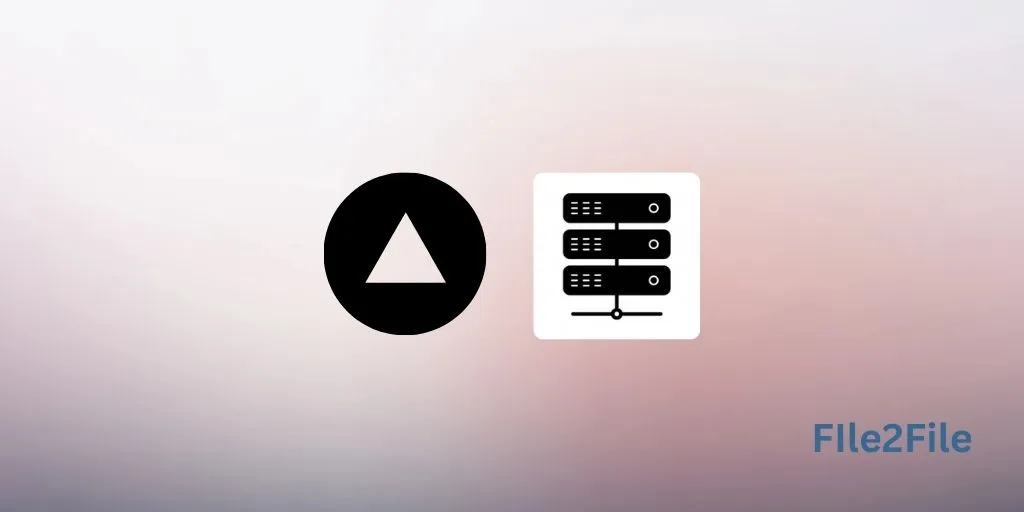
Getting Started with Vercel Hosting: A Step-by-...
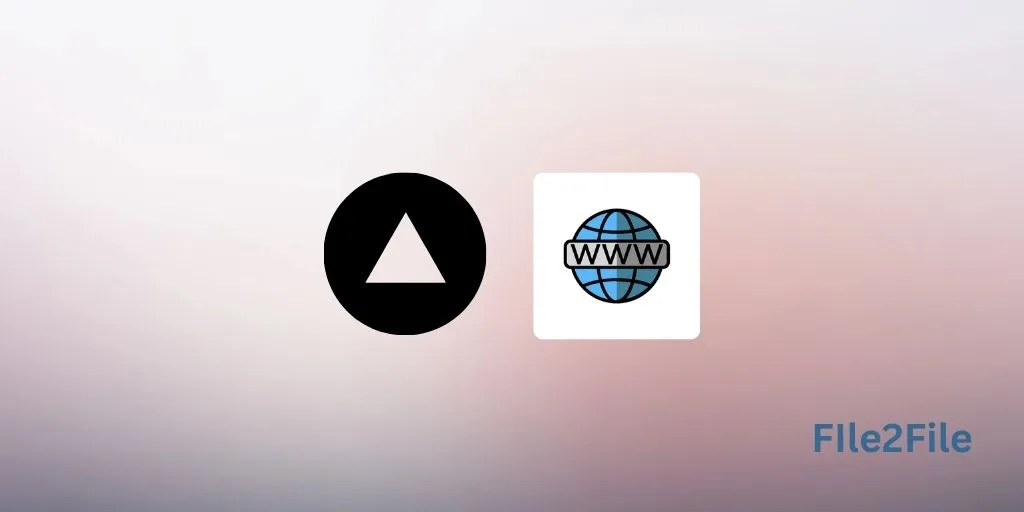
Como Migrar um Aplicativo para Vercel
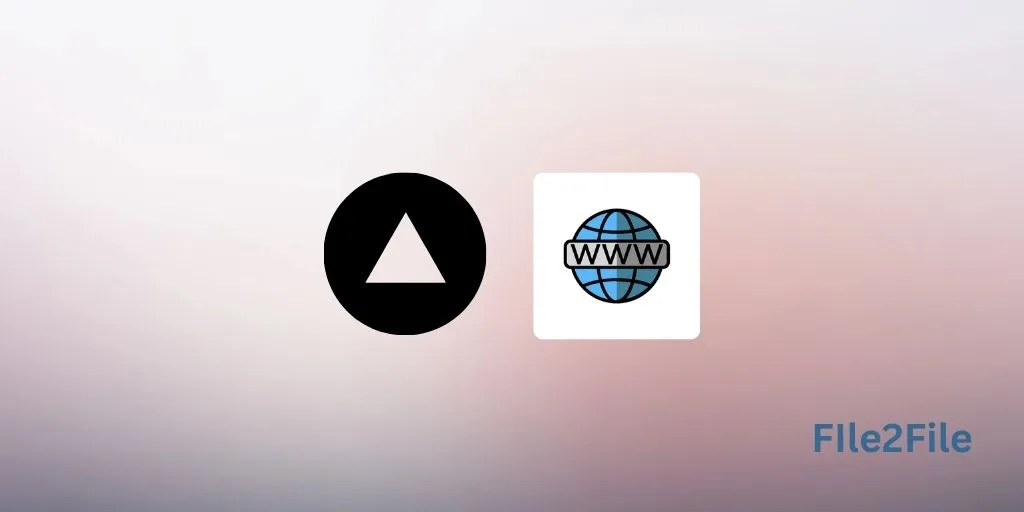
How to Migrate an App to Vercel
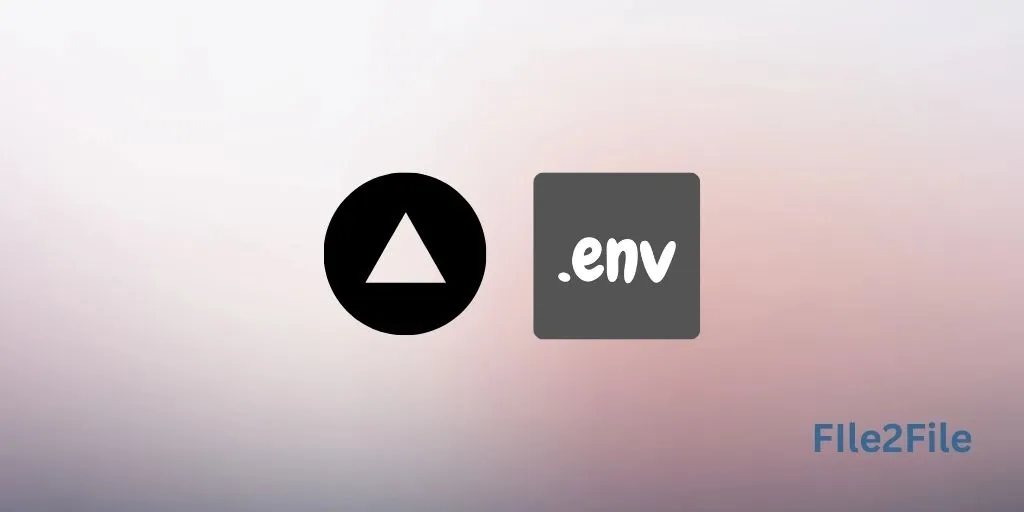
How to Manage Environment Variables on Vercel f...
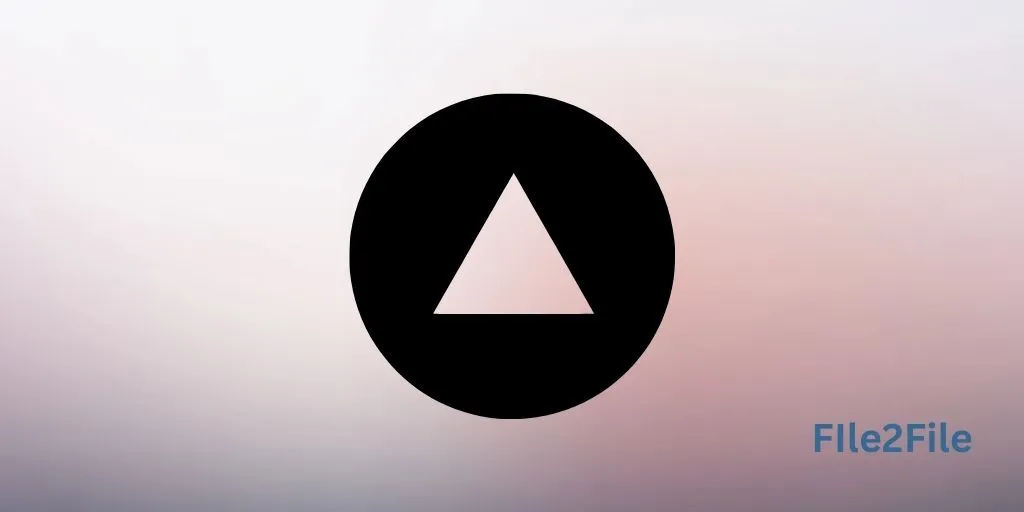